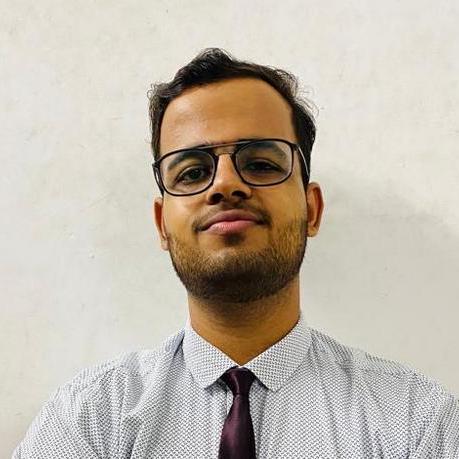
Understanding the Distinction Between nil and Empty Arrays in Ruby
Understanding the Distinction Between nil
and Empty Arrays in Ruby
Introduction
In Ruby programming, distinguishing between nil
and an empty array ([]
) is crucial for writing clean and effective code. This document aims to explore the fundamental differences between nil
and empty arrays, providing insights into when to use each and best practices for handling these cases.
nil
- The Absence of a Value
Definition
nil
represents the absence of a value. When a variable is assigned nil
, it indicates that the variable has not been given a meaningful value.
Use Cases
Unassigned Variables: Variables that have not been assigned any specific value are often initialized to
nil
.Absence of a Result: When a method or operation does not yield a meaningful result, it may return
nil
.
Empty Arrays ([]
) - Intentional Emptiness
Definition
An empty array, denoted by []
, is a collection that intentionally contains no elements. It is a valid instance of the Array
class with a length of 0.
Use Cases
Initialization for Future Elements: If you plan to add elements later, starting with an empty array makes sense.
Representing Empty Collections: When a collection is expected but no elements are present, an empty array serves as a clear representation.
Best Practices
When working with variables that could be nil
or empty arrays, follow these best practices:
Check for
nil
First: Always check if a variable isnil
before attempting any operations specific to arrays.ruby if my_array.nil? # Handle the case when the array is nil elsif my_array.empty? # Handle the case when the array is empty else # Perform operations on the non-empty array end
Use the Appropriate Representation: Choose
nil
when the absence of a value is significant, and use an empty array when intentionally representing an empty collection.Avoid Mixing Types: Ensure that a variable consistently represents either
nil
or an array throughout its lifecycle to prevent unexpected behavior.
Conclusion
Understanding the distinction between nil
and empty arrays is essential for writing robust and readable Ruby code. By applying the best practices outlined in this document, developers can make informed decisions when handling variables that may be nil
or empty arrays, contributing to code clarity and maintainability.
Note: This document provides a basic overview, and further exploration can be done based on specific project requirements and use cases.